NodeJS - The Complete Guide (MVC, REST APIs, GraphQL, Deno)

- Description
- Curriculum
- FAQ
- Notice
- Reviews
Nobles Center doesn’t issue a Certificate for this course, its an affliate program from Udemy.
Node.js is probably THE most popular and modern server-side programming language you can learn these days!
Node.js developers are in high demand and the language is used for everything from traditional web apps with server-side rendered views over REST APIs all the way up to GraphQL APIs and real-time web services. Not to mention its applications in build workflows for projects of all sizes.
This course will teach you all of that! From scratch with zero prior knowledge assumed. Though if you do bring some knowledge, you’ll of course be able to quickly jump into the course modules that are most interesting to you.
Here’s what you’ll learn in this course:
-
Node.js Basics & Basic Core Modules
-
Parsing Requests & Sending Responses
-
Rendering HTML Dynamically (on the Server)
-
Using Express.js
-
Working with Files and generating PDFs on the Server (on-the-fly)
-
File Up- and Download
-
Using the Model-View-Controller (MVC) Pattern
-
Using Node.js with SQL (MySQL) and Sequelize
-
Using Node.js with NoSQL (MongoDB) and Mongoose
-
Working with Sessions & Cookies
-
User Authentication and Authorization
-
Sending E-Mails
-
Validating User Input
-
Data Pagination
-
Handling Payments with Stripe.js
-
Building REST APIs
-
Authentication in REST APIs
-
File Upload in REST APIs
-
Building GraphQL APIs
-
Authentication in GraphQL APIs
-
File Upload in GraphQL APIs
-
Building a Realtime Node.js App with Websockets
-
Automated Testing (Unit Tests)
-
Deploying a Node.js Application
-
Using TypeScript with Node.js
-
Exploring Deno.js
-
And Way More!
Does this look like a lot of content? It certainly is!
This is not a short course but it is the “Complete Guide” on Node.js after all. We’ll dive into a lot of topics and we’ll not just scratch the surface.
We’ll also not just walk through boring theory and some slides. Instead, we’ll build two major projects: An online shop (including checkout + payments) and a blog.
All topics and features of the course will be shown and used in these projects and you’ll therefore learn about them in a realistic environment.
Is this course for you?
If you got no Node.js experience, you’ll love this course because it starts with zero knowledge assumed. It’s the perfect course to become a Node.js developer.
If you got basic Node.js experience, this course is also a perfect match because you can go through the basic modules quickly and you’ll benefit from all the deep dives and advanced topics the course covers.
Are you an advanced Node.js user? Check the curriculum then. Maybe you found no other course that shows how to use SQL with Node.js. Or you’re interested in GraphQL. Chances are, that you’ll get a lot of value out of this course, too!
Prerequisites
-
NO Node.js knowledge is required at all!
-
NO other programming language knowledge (besides JavaScript, see next point) is required
-
Basic JavaScript knowledge is assumed though – you should at least be willing to pick it up whilst going through the course. A JS refresher module exists to bring you up to the latest syntax quickly
-
Basic HTML + CSS knowledge helps but is NOT required
-
1IntroductionVideo lesson
Welcome to this Node.js course! Let me introduce myself and give you a rough overview of this course and what it's all about!
-
2What is Node.js?Video lesson
What is Node.js? That's the most important question in a Node course I'd argue and in this lecture, we'll explore what exactly NodeJS is and why it's amazing.
-
3Join our Online Learning CommunityText lesson
Learning alone is absolutely fine but finding learning partners might be a nice thing, too. Our learning community is a great place to learn and grow together - of course it's 100% free and optional!
-
4Installing Node.js and Creating our First AppVideo lesson
We know what NodeJS is about - let's now see it in action. For that, let's install Node.js and create our first little application in this lecture.
-
5Understanding the Role & Usage of Node.jsVideo lesson
Node.js can be used for a broad variety of things - web servers being the most prominent use-case probably. In this lecture, you'll get an overview of the different things NodeJS can be used for.
-
6Course OutlineVideo lesson
We got a good idea of what Node.js is, now it's time to understand what exactly is in the course. In this lecture, I'll give you a good overview of the course content and the order in which it is presented.
-
7How To Get The Most Out Of The CourseVideo lesson
Your course success matters to me, hence in this lecture, I'll share some best practices regarding the course taking process and how you can get the most out of this course.
-
8Course SetupText lesson
-
9Working with the REPL vs Using FilesVideo lesson
When writing Node code, you got two main options: Files which you execute or the REPL. This lecture explains + explores both alternatives.
-
10Using the Attached Source CodeText lesson
Stuck? Got an error you can't debug on your own? You find snapshots of my code attached to multiple lectures in the course! More information can be found in this lecture.
-
11Module IntroductionVideo lesson
-
12JavaScript in a NutshellVideo lesson
-
13Refreshing the Core SyntaxVideo lesson
-
14let & constVideo lesson
-
15Understanding Arrow FunctionsVideo lesson
-
16Working with Objects, Properties & MethodsVideo lesson
-
17Arrays & Array MethodsVideo lesson
-
18Arrays, Objects & Reference TypesVideo lesson
-
19Understanding Spread & Rest OperatorsVideo lesson
-
20DestructuringVideo lesson
-
21Async Code & PromisesVideo lesson
-
22Template LiteralsText lesson
-
23Wrap UpVideo lesson
-
24Useful Resources & LinksText lesson
-
25Module IntroductionVideo lesson
-
26How The Web WorksVideo lesson
-
27Creating a Node ServerVideo lesson
-
28The Node Lifecycle & Event LoopVideo lesson
-
29Controlling the Node.js ProcessText lesson
-
30Understanding RequestsVideo lesson
-
31Sending ResponsesVideo lesson
-
32Request & Response HeadersText lesson
-
33Routing RequestsVideo lesson
-
34Redirecting RequestsVideo lesson
-
35Parsing Request BodiesVideo lesson
-
36Understanding Event Driven Code ExecutionVideo lesson
-
37Blocking and Non-Blocking CodeVideo lesson
-
38Node.js - Looking Behind the ScenesVideo lesson
-
39Using the Node Modules SystemVideo lesson
-
40Wrap UpVideo lesson
-
41Time to Practice - The BasicsText lesson
-
42Useful Resources & LinksText lesson
-
43Module IntroductionVideo lesson
-
44Understanding NPM ScriptsVideo lesson
-
45Installing 3rd Party PackagesVideo lesson
-
46Global Features vs Core Modules vs Third-Party ModulesText lesson
-
47Using Nodemon for AutorestartsVideo lesson
-
48Global & Local npm PackagesText lesson
-
49Understanding different Error TypesVideo lesson
-
50Finding & Fixing Syntax ErrorsVideo lesson
-
51Dealing with Runtime ErrorsVideo lesson
-
52Logical ErrorsVideo lesson
-
53Using the DebuggerVideo lesson
-
54Restarting the Debugger Automatically After Editing our AppVideo lesson
-
55Debugging Node.js in Visual Studio CodeText lesson
-
56Changing Variables in the Debug ConsoleVideo lesson
-
57Wrap UpVideo lesson
-
58Useful Resources & LinksText lesson
-
59Module IntroductionVideo lesson
-
60What is Express.js?Video lesson
-
61Installing Express.jsVideo lesson
-
62Adding MiddlewareVideo lesson
-
63How Middleware WorksVideo lesson
-
64Express.js - Looking Behind the ScenesVideo lesson
-
65Handling Different RoutesVideo lesson
-
66Time to Practice - Express.jsText lesson
-
67Parsing Incoming RequestsVideo lesson
-
68Limiting Middleware Execution to POST RequestsVideo lesson
-
69Using Express RouterVideo lesson
-
70Adding a 404 Error PageVideo lesson
-
71Filtering PathsVideo lesson
-
72Creating HTML PagesVideo lesson
-
73Serving HTML PagesVideo lesson
-
74Returning a 404 PageVideo lesson
-
75A Hint!Text lesson
-
76Using a Helper Function for NavigationVideo lesson
-
77Styling our PagesVideo lesson
-
78Serving Files StaticallyVideo lesson
-
79Time to Practice - NavigationText lesson
-
80Wrap UpVideo lesson
-
81Useful Resources & LinksText lesson
Productivity Hacks to Get More Done in 2018
— 28 February 2017
- Facebook News Feed Eradicator (free chrome extension) Stay focused by removing your Facebook newsfeed and replacing it with an inspirational quote. Disable the tool anytime you want to see what friends are up to!
- Hide My Inbox (free chrome extension for Gmail) Stay focused by hiding your inbox. Click "show your inbox" at a scheduled time and batch processs everything one go.
- Habitica (free mobile + web app) Gamify your to do list. Treat your life like a game and earn gold goins for getting stuff done!
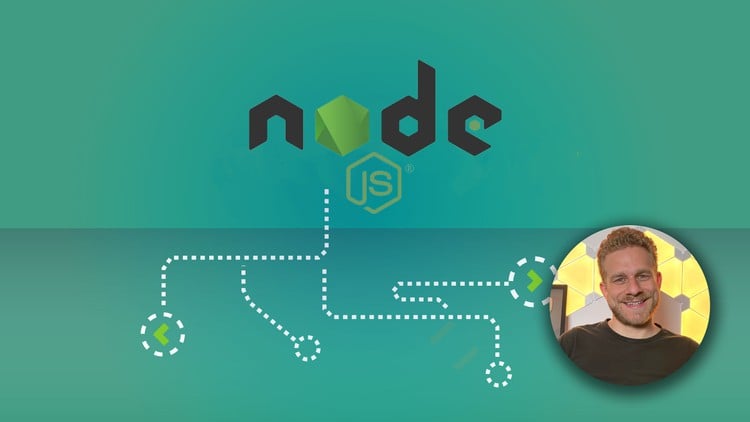
Popular Courses
Archive
Working hours
Monday | 9:30 am - 6.00 pm |
Tuesday | 9:30 am - 6.00 pm |
Wednesday | 9:30 am - 6.00 pm |
Thursday | 9:30 am - 6.00 pm |
Friday | 9:30 am - 5.00 pm |
Saturday | Closed |
Sunday | Closed |